Scripting
Warning: incorrect scripts usage might break everything. Use with caution and always test your scripts!
To make use of scripting system you need to create script file in "Data/Scripts". It's a simple text file with ".script" extension (if you don't have extensions enabled in explorer, then you should do it right now. If you're not willing to, you'll have to find an example script file somewhere and edit it). Script file name can be:
- MapName.script - where "MapName" is... well, name of the map you want to bind this script to.
- DecorationName.script - where "DecorationName" is name of decoration you want to bind this script to. This script will be used when player is near decoration and presses use.
- Any other script will be loaded only if it was set in triggers or terminals settings (see "Triggers")
The scripting language is very simple to use:
Commands are written each on its own line. Any argument these commands need should be separated by a single whitespace. That's it, commands are executed in order top to bottom of the file.
Example:
image Character Sprites/Characters/Character.png
show Character 0 0 2
text "Hello World!"
hide Character 2
timeout 2
map start
This script will load image, set it's name as Character, show it at 0, 0 within 2 seconds, show text on screen, hide image within 2 seconds, set timeout for 2 seconds start the map.
Variables
A variable is a name that you can bind a certain value to. There are three kinds of variables:
- Global - available entire game disregarding of map player currently plays.
- Map variables - available during current map. Deleted after it's over.
- Local variables - available only during current script. Deleted after it's done working.
To set a variable you just need to write it's name and a value, separated by "=". "global." prefix is for global variables, "map." is for map variables:
global.value=10
map.value="Character"
value=10
To use a variable you need to put $ symbol before it's name:
text "$global.value"
image $map.value Sprites/Characters/Character.png
text "$value"
If you need to calculate something (and variable is a number) use next operators:
value+=2 //Addition
value-=2 //Subtraction
value*=2 //Multiplication
value/=2 //Subdivision
value++ //Increment (increase by 1)
value-- //Decrement (decrease by 1)
Be aware that beginning of one variable's name can't contain the full name of the other variable. For example:
global.count=10 global.countnumber=5
It's a wrong way to name variables, because now you can't interact with "global.countnumber" variable - the game engine will always refer to the "global.count" variable first, ignoring the "-number" part on the end. We can easily fix it by changing the letter order:
global.count=10 global.numbercount=5
Conditions
Conditions are blocks of code that will be executed only if some conditions are met. Be aware: "if", and comparator are commands as well, so there MUST be whitespace separating them:
if $value == 1 {
map next
} else {
map goto 3
}
Code inside if block will be executed only if value is 1. Code inside "else" block will be executed if value is NOT 1. Else block may be imotted if you don't need it:
if $value == 1 {
map next
}
Instead of "==" you can use:
- != - condition is met if value is NOT equal
- <= - condition is met if value is less or equal
- >= - condition is met if value is greater or equal
- < - condition is met if value is less
- > - condition is met if value is greater
Procedures
Procedures are pieces of code that can be used whenever you want. Key word "end" marks procedure end, so be sure there is enough of these:
procedure helloworld
text "Hello World!"
end; //semicolon in necessary if you have more than one procedure
procedure helloworld2
text "Hello World 2!"
end
Calling a procedure is just a matter of using command "call":
call helloworld call helloworld2
Procedures exist only inside one single file. If you need them in another file, you'll have to copy them. You can also call procedures from inside other procedures or the very procedure itself. For example:
procedure loop
map.var++
if $map.var == 100 {
map return
} else {
call loop
}
end
This procedure will repeatedly call itself to increment variable "map.var" by one until it would be equal to 100. After that procedure stops the execution of the script.
All procedures must be in the beginning of the script. They wont work when placed after or in the middle of the script.
Commentaries
If you put "//" in front of a line this line will become a commentary - a non-executable code that is just here to set a note for yourself.
//This is a commentary call helloworld
Live Variables in HUD:
Live Variables on screen.
Addin a live variable in to the hud is quite straight forward
Open up the HUD config file and add the following two lines.
The first numeric value is the position on the screen left to right and the second one is vertical position
text 0.01 0.8 (variable name)
variable 0.075 0.8 (global.example)
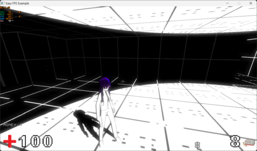
Arithmetic operators: 7 basic math operands are supported,
please note that the engine will not recognize the equivalent long hand form, and uses
Compound assignment.
Also the engine does not allow multiple operations at once, varZ+=$varY++ is
invalid, further more there is no scoping of operations such as (1+2)/3. You may have to get
inventive with creation of temporary variables.
operator | description | example | equivalent |
---|---|---|---|
+= | Addition | var+=$number | var=$var+$number |
-= | Subtraction | var-=$number | var=$var-$number |
*= | Multiplication | var*=$number | var=$var*$number |
/= | Subdivision | var/=$number | var=$var/$number |
%= | Modulo operand | var%=$number | var=$var%$number |
++ | Increment (add 1) | var++ | var=$var+1 |
-- | Decrement (subtract 1) | var-- | var=$var-1 |
Advanced Arithmetic builtin commands:
command | description | example | Version of efpse |
---|---|---|---|
SIN | sin | varX=SIN($varY) | 1.11 alpha test 1 |
COS | cosine | varX=COS($varY) | 1.11 alpha test 1 |
TAN | tangent | varX=TAN($varY) | 1.11 alpha test 1 |
ASIN | arc sine | varX=ASIN($varY) | 1.11 alpha test 1 |
ACOS | arc cosine | varX=ACOS($varY) | 1.11 alpha test 1 |
ATAN | arc tangent | varX=ATAN($varY) | 1.11 alpha test 1 |
ATAN2 | arch tangent2 | varX=ATAN2($varY) | 1.11 alpha test 1 |
POWER | power of | varX=POWER($varY) | 1.11 alpha test 1 |
SQRT | Square root | varX=SQRT($varY) | 1.11 alpha test 1 |
ABS | absolute value, ie -1 becomes 1 | varX=ABS($varY) | 1.11 alpha test 1 |
FLOOR | down to integer | varX=FLOOT($varY) | 1.11 alpha test 1 |
CEIL | up to next integer | varX=CEIL($varY) | 1.11 alpha test 1 |
CLAMP | clamp | varX=CLAMP($varY) | 1.11 alpha test 1 |
MIN | min | varX=MIN($varY,$varZ) | 1.11 alpha test 1 |
MAX | max | varX=MAX($varY,$varZ) | 1.11 alpha test 1 |
ROUND | rounds to nearest 10th | varX=ROUND($varY) | 1.11 alpha test 1 |
RANDOM | random number between (min,max) | varX=RANDOM(0,3) | stable pre-CE |
Generic commands list:
Please note that rectangular braces are here just to mark an argument. You do not need to put them yourself.
auto
auto [0/1] - sets auto execution. By default script is executed line-by-line as player presses left mouse button or use button.
timeout
timeout time - sets timeout. Next script line will be executed automatically when timeout is over.
call
call procedureName - calls a procedure.
pause
pause - pauses script until mouse button is pressed or a use key is pressed.
halt
halt - stops script entirely.
keeptrigger
keeptrigger - prevents trigger that called the script from being deleted when the script ends.
Game commands list:
map
map start [keepmusic] - starts map after cutscene script is over. Must only be used in cutscenes at the start of a map! If keepmusic is 1 - music played in the cutscene will be played on map as well. If it's 0 or omitted, map music will be played.
map next - moves to the next map.
map return [KeepSounds? 0/1/2/3] - return to map. Use only in triggers, terminals or active decorations with scripts. KeepSounds: 0 stops all sounds, 1 keeps music, 2 keeps music and sfx , 3 keeps scripted sounds
map quickreturn [KeepSounds? 0/1/2/3] - return to map without interrupting player input, enabling keybind scripts to run repeatedly while held, and avoiding input delay when a trigger is activated
map goto [index] - moves to a certain map. index - map number in the list (starting from 0).
experimental ability to run a looping script in the background.
If you have a mapname_loop.script, it will run continuously in the background while you're on that map. For now, I don't recommend using this in any games you're about to release. It's more like a public beta test. This feature may be changed, or run at a fixed time interval in a future update.
Player and entity commands | |
---|---|
player |
player
player heal [amount] - heals player. player hurt [amount] - hurts player. player teleport [tileX] [tileY] [tileZ] [relative? 0/1] - moves player to tile coordinates (0 to 63). tileY is floor number (0 to 8). TileX and tileZ can be seen in the editor. If relative is set to 1, the destination will be relative to the player's current position player move [offsetX] [offsetY] [offsetZ] - shifts player to a certain number of units. player rotation [angleX] [angleY] - sets camera rotation. angleX is up-down rotation (0 - front, 90 - up). angleY is sideways (0 is to the right, 90 is up on the map) player retro [0/1] - if 1, deactivates player and weapon viewbob. player turn [0/1] - if 1, deactivates mouse usage and strafing. player steps [0/1] - if 0, deactivates footsteps sound. player speed [value] - changes player speed. player sethp [value] - directly set the player's hp without triggering flashes. player setmaxhp [value] - set the player's max hp. player givearmour [value] - gives armour to the player.. player takearmour [value] - takes armour from the player. player setarmour [value] - set the player's armour. player setmaxarmour [value] - set the player's max armour. player cancrouch [0/1] - set whether the player can crouch. player canjump [0/1] - set whether the player can jump. player height [walk/crouch] [value] - set the player's walk or crouch height. Default walk height is 42. Default crouch height is 25.2 player weapon holster - holsters the current weapon without removing it player zoom [value] - Sets the zoom/fov to use (0 = normal, 100 = max zoom, -100 = min zoom) player camspeed [value] - Sets the multiplier to use for camera turning speed (1 = normal, 0.5 = half, etc) player check hp [variable] - sets variable to the player's current health. player check maxhp [variable] - sets variable to the player's max health. player check armour [variable] - sets variable to the player's current armour. player check maxarmour [variable] - sets variable to the player's max armour. player check key [number] [variable] - sets variable to 1 or 0, indicating if the player has key[number]. player check weapon [slot] [variable] - sets variable to 1 or 0, indicating if the player has weapon[slot]. player check ammo [slot] [variable] - sets variable to the total amount of ammo in weapon[slot]. player check mag [slot] [variable] - sets variable to the amount of ammo in weapon[slot]'s mag. player check heldweapon [variable] - sets variable to the currently selected weapon slot. player check position [varX] [varY] [varZ] - sets variable x/y/z to the player's current position. player check rotation [varX] [varY] - sets variable x/y to the player's current x/y rotation. (y-axis rotation is for player velocity [set/add] [x] [y] [z] player jumpheight [$height]" (default is 256). |
entity |
entity delete [tileX] [tileY] [tileZ] - deletes entity in a certain tile. tileZ is floor number.
entity delete me - deletes entity that called a script (or decoration that player used). entity move [tileX] [tileY] [tileZ] [offsetX] [offsetY] [offsetZ] - shifts entity. entity spawnat [enemyname/decorationname/Key1-3/Hp1-3] [tileX] [tileY] [tileZ] [Rotation] - creates entity at tile coordinates (as shown in map editor). entity spawnatpos [enemyname/decorationname/Key1-3/Hp1-3] [X] [Y] [Z] - creates entity at a certain number of units. |
door |
door open [tileX] [tileY] [tileZ] [stay? 0/1] - opens a door in a tile. If stay is set to 1, the door will only close again with a script.
door close [tileX] [tileY] [tileZ] - closes a door. door lock [tileX] [tileY] [tileZ] - locks a door. It will not open by player ever, only through script. door unlock [tileX] [tileY] [tileZ] - unlocks a door. |
light and world |
light create [tileX] [tileY] [tileZ] [radius] [r] [g] [b] - creates a light source at tile coordinates (as shown in map editor) with your own preferences.
light move [tileX] [tileY] [tileZ] [offsetX] [offsetY] [offsetZ] - shifts any light source. light status [on/off] [tileX] [tileY] [tileZ] - turns on/off any light source. light offset [x] [y] [z] (moves light without updating its tile position, allowing lights to be moved around without needing to recalculate the new position) flashlight state [0/1] flashlight lock [0/1] flashlight range [range] flashlight colour [r] [g] [b] flashlight radius [radius] light ambient [r] [g] [b] [a] light sun colour [r] [g] [b] [a] light sun direction [x] [y] [z] skybox texture Path/To/Texture.png fog colour [r] [g] [b] fog distance [dist] shader texture [uniformName] [Path/To/Texture.png] shader bool [uniformName] [0/1 or true/false] |
status |
status [string] [time] - shows a text for a certain amount of time. |
give |
give weapon [slot] - gives weapon to a slot (1 - 8).
give ammo [slot] [amount] - gives ammo for a slot. give key [number] - gives key to the player. |
take |
take weapon [slot] - takes weapon from a slot (1 - 8).
take ammo [slot] - takes ALL ammo from a slot. take key [number] - takes key from player. |
cursor |
cursor [1/0] - shows or hides a cursor. Not supposed to work while showing text with "vn" on. |
shader |
shader set [name] - sets current shader. Default shader name is - "default".
shader set [name] [path] - loads a shader, sets it's name and sets it as a current one (vert and frag shaders should be placed in Data/Shaders). |
game |
game save slot [1-8] - saves game to a slot.
game save auto - saves game to autosave slot. game load slot [1-8] - loads game from a slot. game load auto - loads game from autosave slot. |
gravity |
gravity [strength] - sets the strength of the gravity (default is 970) |
weapon |
weapon maxammo [slot] [value] - sets the max ammo of weapon[slot] to [value]
weapon magsize [slot] [value] - sets the max magazine size of weapon[slot] to [value] weapon damage [slot] [value] - sets the damage of weapon[slot] to [value] weapon firerate [slot] [value] - sets the fire rate of weapon[slot] to [value] weapon bullets [slot] [value] - sets the bullet count of weapon[slot] to [value] weapon reloadspeed [slot] [value] - sets the reload speed weapon[slot] to [value] weapon projectilespeed [slot] [value] - sets the projectile speed of weapon[slot] to [value] weapon explosion [slot] [value] - sets the explosion radius of weapon[slot] to [value] weapon recoil [slot] [value] - sets the recoil of weapon[slot] to [value] weapon spread [slot] [value] - sets the spread of weapon[slot] to [value] weapon recoilrecovery [slot] [value] - sets the recoil recovery of weapon[slot] to [value] |
HUD |
Animated Hud Examplehud image [imageName] [x] [y] [scale] [path] [layer] - Creates or updates a hud image. Using the same imageName will replace the previous hud image with that name. Higher layer images will be rendered on top of lower-layer images. Calling 'hud image [imageName]' without any other parameters will remove the specified hud image.
hud autoscale [image/mask name] [stretch/width/height/off] |
Settings | check resolution [resX] [resY] |
Cutscene commands list: | |
---|---|
vn |
vn [1/0] - enables or disables visual novel mode. |
vn speed | (text string [r] [g] [b] [characters per second, default is 80]). |
text |
text [string] [r] [g] [b] - places text on screen (or in a textbox of VN mode is enabled) with a set color (if omitted color will be white). |
font |
font [size] - sets font size |
# | Using the # hashtag symbol in a text string will tell the engine to move everything after the # on to the next line below. This is how you break up longer text to keep it on the screen. |
preload |
preload [1/0] - enables or disables images preloading. Disable to save memory. Recommended on long cutscenes. |
image |
image [name] [path] - loads an image and sets it's name. Path may be anything in scope of your project folder (omit ProjectName/ part. For example "Images/Image.png" will tell engine to look into "ProjectName/Images/Image.png") |
sound |
sound [name] [path] - loads sound and sets it's name. Path rules are the same as for images. |
show |
show [imageName] [x] [y] [time] - shows image within set time. imageName is the name that was set to an image when loading. If time is 0 or omitted then image will be shown immediately. |
bg |
bg [imageName] [time] - shows background within set time. Unlike images bgs are stretched to fill entire screen. |
hide |
hide [imageName] [time] - hides image within set time. Including backgrounds and buttons. imageName is name of image that was set when loading. |
play |
play sound [name] - plays sound with a name that was set when loading.
play music [path] - plays music. Path rules are the same as for sounds and images. play video [path] - plays video. Currently only supports efpse .vid files. |
stop |
stop music - stops cutscene music. You can't stop map music.
stop sound [name] - stop the specified sound. stop sounds - stop all played sounds. |
button |
button [imageName] [x] [y] [procedurename] - shows button that will call procedure upon clicking on it. Button image should consist of three images in a row. The first one is for button when it's not pressed and cursor is not over it. Second is when cursor is over it. Third - when it's pressed. |
label |
label imageName [x] [y] [string] [size] [r] [g] [b] [center/left/right] - places text on any place of the screen. |
move |
move [imageName] [x] [y] [time] - moves image to certain coordinates within time. |
front |
front [imageName] - moves image in front of everything else. |
back |
back [imageName] - moves image to the back.
There is also exactly one special command that is used only in menu script: |
bind |
bind [key] [scriptname] - bind a script that will be executed when a keyboard button is pressed. |
unbind | unbind [key] |